Published: 2024-07-06
Lesson 2b - Collections
Time To Complete: 1.5 hours
Lists, Tuples, and Dictionaries
Lists are Powerful
Lists are data structures. Used to store things.
- Lists are Dynamic Arrays.
- An array is a collection of elements.
- Each element is identified by a key, which is called an “index”.
A list is created using[]
.
Examplemy_list = []
.
Let's make a grocery list.
food_list = [
'bread',
'butter',
'milk',
'eggs',
'cheese'
]
print(food_list)
Lists are powerful because we can manipulate the list itself, and the elements within it.
note: elements are like items
We can print a specific element by using the item’s position or index.
print(food_list[2])
Hang on, why use 2 instead of 3? How did using 2 return the third item?
Lists start at index position 0. Meaning that the first item in a list is position 0.
Try it out.
food_list[0]
It prints the first item, bread.
You can also change items within a list easily.
#I'm actually lactose intolerant...
food_list[2] = 'oat milk'
print(food_list)
You have replaced position 2, milk, with oat milk.
Using lists you can also show a sublist, a specific range of items within that list.
print(food_list[1:4])
The above code tells python, “show the items from index 1 up to (but not including, because sublists are exclusive) index 4.” In other words return items at index 1, 2, 3.
Items at index 0 and 4 will not be shown.
Play around with using different ranges to get a feel for how slicing works, and how indexing works.
Lists can be used to store all sorts of items, like numbers
nums = [1,3,5,7,9,11]
nums[1:4]
nums[3]
nums[-1] #print the last item.
Lists can hold strings.
words = ['hello', 'hi', 'hola', 'gutentag']
You can even mix up items in a list. Use multiple data types.
nums = [1,3,5,7,9,11]
words = ['hello', 'hi', 'hola', 'gutentag']
list = [nums, words]
print(list)
We combined the two lists as a 2D list!
These different data types can also be stored as a single list.
mixed_list = ['one',3,'five',7,'nine',11]
print(mixed_list)
Adding items to a List
How do we add new items to an existing list? We use the append function.
Function
: Function is a chunk of code that tells python to do something.
food_list.append('Bagel')
print(food_list)
You can add multiple items this way.
Try playing around with the append function. Add 3 more items to the shopping list.
Removing Items From a List
To quickly remove items from a list, in python we use the del command.
Remove the last item from your list, then the third.
del food_list[-1]
del food_list[2]
NOTE: Remember that positions start at zero, so food_list[2] actually refers to the third item in the list. Trick: You can use [-1] to find the last item in a list.
List Arithmetic
You can join lists by adding them just like numbers using the +
sign.
greeting_num = [1,2,3,4]
greetings = ['hello', 'hi', 'hola', 'gutentag']
greeting_list = greeting_num + greetings
print(greeting_list)
Alternatively, you can use print(greeting_num + greetings)
and you will get the same result.
You can also multiply lists using *
nums = [1,2,4,7,5]
print(nums * 2)
Notice how python did not multiply each value inside of the list by two, but instead return 2 copies of the list as one , one-dimensional list.
Python does not support using subtraction(-)
or division(/)
for list arithmetics.
You cannot add something to a list that isn’t a list with +
.
This will result in an error.
list1 = [2,3]
list1 + 50
In all cases an error occurs because there are too many possibilities when you try to divide or subtract lists, or add things to a list that isn’t a list.
Tuples
Tuples are a like lists, but different.
- They are not mutable, they cannot be changed.
- Defined using “()”
- Python reads them faster.
fib = (1,3,4,1,2,2,1)
print(fib[5])
If you try to replace an item inside of a tuple, you get an error.
If you want your tuple to be different, you need to remove or delete it and start again.
Why would you use one then? Tuples are immutable, meaning they cannot be changed or manipulated. Tuples are an array with set size and set values. Python can read tuples faster than lists. There are some situations in programming were it may be advantageous to have a tuple that can read and grab data really quickly.
Dictionaries (maps)
A map, or dictionary, or hashmap is a data structure that organizes a collection of items as a key-value pair.
Each item in the dictionary has a key and a value. The key is the location, the value is mapped to a location(key).
If there is a dictionary or map of a bunch of people’s favorite sport, we can easily lookup someone’s favorite sport rather than skimming a long list of each person’s favorite sport.
favorite_sports = {'Ralph' : 'Football',
'Michael' : 'Basketball',
'Edward' : 'Baseball',
'Rebecca' : 'Netball',
'Ethel' : 'Badminton',
'Frank' : 'Rugby'}
print("\nFavorite Sport:")
print("Michael's: " + favorite_sports['Michael'], "\nEthel's: " + favorite_sports['Ethel'])
Trick: the \n
is an escape character that simply tells python to move the string that follows it to the next line.
Building a dictionary:
- wrap the key-value pairs with
{}
- Use colons to separate each key from its value
- separate each entry with a
,
In thefavorite sports
dictionary the key and the value are strings. Keys and values can also be integers, or other data types.
Adding values
To add an entry to the dictionary we can use the update function and pass in a new key-value pair.
favorite_sports.update({"Jake": "Basketball"})
print(favorite_sports)
Deleting values
To delete value, simply use the del
command and provide the dictionary key.
del favorite_sports['Jake']
print(favorite_sports)
Change a Value
You can change a value by using the key
favorite_sports['Ralph'] = 'Ice Hockey'
print(favorite_sports)
Dictionaries work a lot like lists and tuples, except you cannot use operators to combine dictionaries like you can lists.
Python doesn’t know how to handle ordering when you try to smash different dictionaries together. Instead use the update()
function used earlier.
Bio
Create a dictionary for yourself.
- You can map lists to keys to hold multiple values in one key. *
Jake = {
'name': "Mr.Neeley",
'favorite foods': ['Pizza','Chicken Tikka Masala','French Fries'],
'hobbies': ['photography','programming','reading','video games','music']
}
Add more than 3 categories.
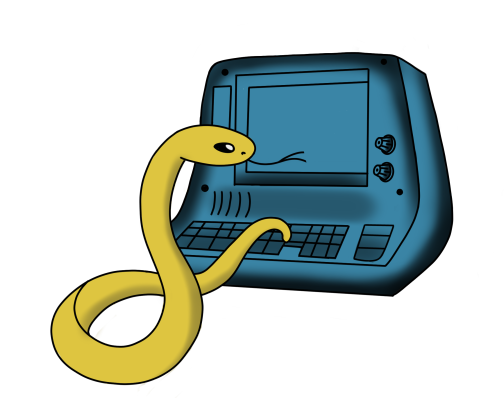