Published: 2024-06-29
Lesson 2a - Conditions
Time To Complete: 2 hours
Asking Questions
IF STATEMENT
It is common to ask questions in programming. Such as “is x greater than y?”
x = 4
y = 2
if x > y:
print("yes, x is greater than y")
Conditions Help Us Compare Things
A condition is a programming statement that compares things tells us whether the comparison evaluates to either True(yes) or False(no).
x = 11
if x > 10:
print(x)
This is simply a way of asking “is the value of ‘x’ greater than 10?”
hair_color = "brown"
if hair_color == "brown":
print(hair_color)
if hair_color == "dark brown":
print(hair_color)
when asking if a value is equal to another value we have to use ”==“.
”=” is reserved for assigning variables. ”==” checks to see if two values or variables are equal.
age = 9
if age < 10:
print("you are too young for this course...")
what happens if age is greater than 10?
age = 11
if age < 10:
print("you are too young for this course...")
Nothing happens.
The print statement only runs if the value of age is less than 10.
Let’s change the if statement to use the greater-than-or-equal(>=) condition.
age = 11
if age <= 10:
print("you are too young for this course...")
Block
An if statement is made up of
- if
- condition
- then a (:) followed by a block of code that runs if the condition is true
A block of code is a grouped set of programming statements.
x = 4
y = 2
print("is x greater than y?")
if x > y:
print(x>y)
print("yes, x is greater than y!")
The block of code above only runs if the condition is true.
Blocks are necessary because they tell python what comes next if we ask a question using an “if” statement.
This means that blank spaces or whitespaces in python are important.
x = 4
y = 2
print("is x greater than y?")
if x > y:
print(x>y)
print("yes, x is greater than y!")
The code above will cause the SyntaxError: unexpected indent
to be thrown.
Python only expects indents when there is a some condition followed by a colon (:) then a block of code.
x=10
y=5
if x>y: #block 1
print(x>y) #block 2
#not negative
if x > 0 and y > 0:
print("x and y are positive!") #block 3
print("yes, x is greater than y!")
If you run the above, all conditions return true and all 3 blocks run. What happens we change x and y to x=-2 and y=-4?
x=-2
y=-4
if x>y: #block 1
print(x>y) #block 2
#not negative
if x > 0 and y > 0:
print("x and y are positive!") #block 3
print("yes, x is greater than y!")
The first condition in block 1 returns true so the code in block 2 will run.
The second condition in block 2 returns false so the code in block 3 will NOT run.
Python skips block 3 and runs the last line in block 2, then exits.
IF-THEN-ELSE
If statements can be used to the computer when to do something when a condition is met (true).
You can also use if statements to do something when a condition is not met(false).
age = 11
if age < 10:
print("you are too young for this course...")
else:
print("welcome to the course!")
The code above will run the second print statement, the “else” condition.
Now change age to 9.
age = 9
if age < 10:
print("you are too young for this course...")
else:
print("welcome to the course!")
You should see the first print statement run.
If you don’t make sure your if statement reads as follows if age < 10:
.
IF and ELIF Statements
We can ask additional questions by including additional conditions using elif(else if).
Let’s write some code to make sure a user is old enough to take our course.
The user’s age should be non-zero and non-negative(positive).
age = int(input("enter your age: "))
if age > 0:
if age < 10:
print("you are too young for this course...")
elif age >= 10:
print("Welcome to the course!")
else:
print("invalid input")
The above code checks to see if the age entered by the user is valid (greater than 0) then checks to see if the user age is less than 10 then prints that the user is too young. Else if, the user is 10 or older then prints that the user is welcome to the course, else the user’s input was NOT valid (a value less than 1 was entered).
why “if age > 0” and not “if age > 1”?
Good catch, but if you use “if age > 1:” and you enter “1” you would expect the “too young” message, but instead you get “invalid input.” huh?
This is because you don’t have a condition to handle “1”, because the code was written in a way that python expects values greater than 1 or it is invalid. This is because using <
or >
is exclusive.
To fix this, you just use the following if age >= 1:
which is inclusive.
Now, if 1 is entered the system will declare the user is too young as EXPECTED.
Alternatively we can go back to using age > 0:
.
How? using age > 0 if you enter 1 we get the “too young” message as expected and if you enter 0 you get invalid as expected.
It is technically possible to be 0 years old (newborn), but for this example we will declare that in order for an age to be valid, it must be non-zero and non-negative.
Ultimately, the way you write your conditions depends on context. The design of your app or your program may require that age = 0 be valid, it may not. It’s up to you or your future boss.
Combining Conditions
You can combine conditions by using the keywords and
and or
, which
produces shorter and simpler code.
OR
if age == 11 or age == 12 or age == 13 or age == 14:
print("you're likely a middle schooler")
elif age < 11:
print("you're likely a elementary schooler")
elif age < 19:
print("you're a highschooler")
else:
print("you're something else...")
In this code above, if any of the conditions are true the code in the first if statement, the code in the first if block will run, elif your age is less than 11 the code in the that elif block will run, elif your age is less than 19 the code in that elif block will run, else none of those conditions are true you’re an adult i.e something else.
This code has some issues though. If you set age equal to 2, the program will run the code inside the first elif block. What 2 year old is an elementary student? A Genius I suppose. If you enter a goofy number like 11.5(eleven and a half years old) our program will think the user is likely a highschooler. Also, what happens if you set the age equal to 15? The code in the second elif block will run as expected. It is likely a 15 year old would be in highschool, but to anyone else reading this code it may not be clear. That is an issue.
In this example these issues can be solved by use ‘and.’
It tightens up our code, making conditions a little more specific.
It also makes it easier to understand.
AND
age=15
if age == 11 or age == 12 or age == 13 or age == 14:
print("you're likely a middle schooler")
elif age >= 15 and age < 19:
print("you're a highschooler")
elif age >= 5 and age < 11:
print("you're likely a elementary schooler")
else:
print("you're something else...")
Ninjas
Write a program that can assess how many ninjas you can fight. If the amount of ninjas is less than 30, print “I think I can take them.” If the amount is 10 or less, print “I can take those ninjas.” Else, print “There’s too many of them. I need backup.”
test with ninjas = 5, 10, 12, 30 and 50.
ninjas = 5
if ninjas > 10 and ninjas <= 30:
print("I think I can take them.")
elif ninjas <= 10:
print("I can take these ninjas")
else:
print("There's too many of them. I need backup.")
Twinkies
Write a program that checks whether the number of Twinkies is less than 100 and if true, prints “too few.” If the amount is greater than 500 and if true, prints “too many”. If the amount is between 100 and 500 print “just enough.”
twinkies = int(input("Enter number of twinkies: "))
#code the rest
Just the Right Number
You find a wallet on the street. Create a program that checks whether the amount of money contained in the variable wallet
is between 100 and 500 OR between 1000 and 5000. Then check if the amount is between 100 and 500, if true print “wow, it’s my lucky day” OTHERWISE, check if the amount is between 1000 and 5000, if true print “This is a very large amount of money. I should probably report this as lost…“. Else you leave the wallet alone and walk away.
print("You find a wallet on the street. You pick it up...)
wallet = int(input("Enter the amount of money found in the wallet: "))
#code the rest
Solutions can be found at the end of this document.
Don’t cheat yourself try coding on your own first.
Note: there can be multiple solutions
Solutions
Twinkies
#solution 1
#Test with 200.
twinkies = int(input("Enter number of twinkies: "))
if twinkies < 100:
print("too few...")
elif twinkies >= 100 and twinkies <= 500:
print("just enough")
elif twinkies > 500:
print("too many...")
else:
print("invalid")
#Output: "just enough"
or
#solution 2
twinkies = int(input("Enter number of twinkies: "))
if twinkies < 100:
print("too few...")
elif twinkies >= 100 and twinkies <= 500:
print("just enough")
else:
print("too many...")
Just the Right Number
#example solution:
print("You find a wallet on the street. You pick it up...)
wallet = int(input("Enter the amount of money found in the wallet: "))
if wallet >= 100 and wallet <= 500 or wallet >= 1000 and wallet <= 5000:
if wallet >= 100 and wallet <= 500:
print("wow, its my lucky day")
elif wallet >= 1000 and wallet <= 5000:
print("This is a very large amount of money. I should probably report this as lost...")
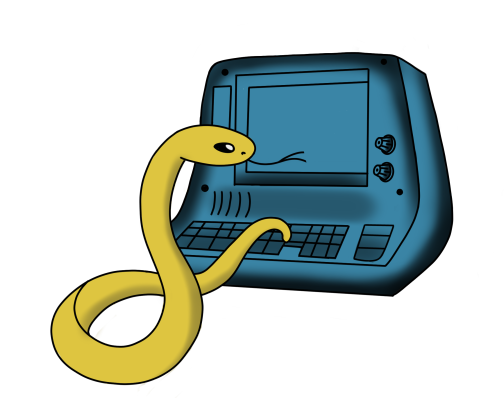