Published: 2024-07-20
Lesson 4 - Writing Programs
Time To Complete: 2 hours
The goal of this lesson is to write a program using all of the concepts learned from lessons 1-3.
You will build:
- A Simple Calculator Program
Simple Calculator Program
The goal of this Program is to provide a user with a simple calculator program that can compute addition, subtraction, multiplication and division.
The Algorithm:
- The Program will give the user a list of choices
- Addition
- Subtraction
- Multiplication
- Subtraction
- The user will select an operation
- The user will enter 2 numbers as inputs
- The Program will compute the result of the two inputs based on the operation selected
- The Program will allow the user to start a new calculation after a value has been calculated
- giving the user the the following prompt
Quit(y/n)?
as an input n
restarts the program,y
closes the program.
- giving the user the the following prompt
- The program should handle ‘ValueErrors’ and “bad” inputs
- i.e. providing an error message if the user enters a
string
instead of anint
without the program crashing.
- i.e. providing an error message if the user enters a
Code:
Writing The Operation Logic
Before any code is written, it’s good to take a second and break down the big problem into smaller problems. This can be done by thinking about isolating each task by either tackling the problem one item at a time or by drawing out some solutions.
Let’s start with the first 2 bullets:
- The Program will give the user a list of choices
- Addition
- Subtraction
- Multiplication
- Division
- The user will select an operation
print("Operations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
user_input = input("Make a Selection: ")
In the above code, a list of operations will print out in the console and the user can provide an input. Currently nothing will happen. Let’s print user_input
to quickly test the code.
Add:
print(user_input)
and run the code.
The program will run, print the list of operations and print “Make a Selection” and wait for an input to be provided. You can type in anything, hit enter, and the program will print what you entered.
In python, the input function allows for user input. Python will stop and wait for an input to be entered before proceeding.
Notice how in the list of operations numbers were provided? Those numbers will be used to select an operation. If a user wants to perform addition, they will enter 1
as the input. Let’s write some conditions to allow the user to select which operation they would like to perform and then return their selection.
We will start with addition first:
if user_input == 1:
print(user_input + ". Addition")
Great, now let’s test it.
Nothing happened. How come 1. Addition
didn’t print?
By default the input()
accepts a value, the value we place inside of the ()
, as a string. The condition to check if the user enters a 1
for addition is checking if the user_input == 1
. Since the input()
accepts a value as a string the user_input == 1
evaluated as false because 1
was entered as an string and if user_input == 1:
is checking if the input is an integer of value 1 not “1”. Remember strings and integers are different.
How can this be fixed? Simple, you can cast the input()
function as a integer like so
user_input = int(input("Make a Selection: "))
This will instruct python to read the input as an integer. Now, when 1
is entered as the input, the user_input == 1
condition will evaluate to true, but the print(user_input + ". Addition")
will not work just yet. In order to print the user input and the “Addition” string as one string, we must cast the user_input
back to a str like so print(str(user_input) + ". Addition")
.
Now the remaining conditions can be added.
elif user_input == 2:
print(str(user_input) + ". Subtraction")
elif user_input == 3:
print(str(user_input) + ". Multiplication")
elif user_input == 4:
print(str(user_input) + ". Division")
Be sure to test each.
Calculating Values
Moving on, the next steps are:
- The user will enter 2 numbers as inputs
- The program will compute the result of the two inputs based on the operation selected
First, let’s tackle how the users will enter two numbers for calculation.
if user_input == 1:
print(str(selection) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
Using the addition condition as an example, x
and y
will be declared and they will hold two input functions as integers, the first number and the second number. If you run the code and test using the addition operation, python will ask you to input the first number and the second. Our code won’t calculate the two values yet. Let’s fix that.
if user_input == 1:
print(str(selection) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " + " + str(y) + " = " + str(x + y))
To print the result out nicely, a string will be built to print the first value, the second and the result of adding the two together. Since the output will be a string the integers must be cast as strings.
Now the remaining conditions can be completed.
print("Operations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
user_input = int(input("Make a Selection: "))
if user_input == 1:
print(str(user_input) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " + " + str(y) + " = " + str(x + y))
if user_input == 2:
print(str(user_input) + ". Subtraction")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " - " + str(y) + " = " + str(x - y))
if user_input == 3:
print(str(user_input) + ". Multiplication")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " * " + str(y) + " = " + str(x * y))
if user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
So far your code should look like the sample above.
Be sure to test your code.
Running Code Without Restarting
Currently, our program isn’t very re-usable. The program closes after every calculation. How can we make the user experience better?
Refer back to written algorithm:
- The program will allow the user to start a new calculation after a value has been calculated
- giving the user the the following prompt
Quit(y/n)?
as an input n
restarts the program,y
closes the program.
- giving the user the the following prompt
In lesson 3, we explored a particular kind of loop that allows a block of code to be executed over and over again until a specific condition was met. Do you remember what that loop was called?
A while
loop! To make the program run continuously we will place all of our calculation logic inside of a while
loop. After a calculation has been performed the program will ask the user if they wish to Quit(y/n)?
. If the user inputs y
for ‘yes’ the while
loop will break. If the user enters n
for ‘no’, then the while
loop will continue.
quit = "n"
while quit == "n":
First we will create the variable quit
which will hold the users choice. At the start of the program it will be set to ‘n’ because we don’t want python to quit before the user can provide any data.
To execute our program continuously, place all of the code that has already been written inside of the while quit == "n":
. As long as quit == n
is true, our calculator will reset after each calculation.
Now the code will look like this:
print("Operations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
quit = 'n'
while quit == 'n':
user_input = int(input("Make a Selection: "))
if user_input == 1:
print(str(user_input) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " + " + str(y) + " = " + str(x + y))
elif user_input == 2:
print(str(user_input) + ". Subtraction")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " - " + str(y) + " = " + str(x - y))
elif user_input == 3:
print(str(user_input) + ". Multiplication")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " * " + str(y) + " = " + str(x * y))
elif user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
If you run the code as is, it will run infinitely. This is called an infinite loop. In most cases this is very bad. An infinite loop occurs when no condition to break the loop has been provided. Fortunately for us, because our program has to stop and wait for inputs its not so bad. In other cases an infinite loop can crash a server or a computer. It is always import to review your code to make sure you have a condition that will end your while loop before you test it.
The infinite loop can be fixed by added an input for quit
at the end of the loop.
#the division condition at the end of the loop
elif user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
quit = input("Quit(y/n)? ")
After each calculation the program will ask the user if they wish to quit and python will record their response and store it in the quit
variable we created at the start of the program. If the user enters ‘y’ it will replace the value that quit
is already holding and when the loop starts over it will check while quit == 'n':
again and evaluate to false because quit = "y"
. Python will skip over the code in the while
loop and close the program because there is no code outside of the while
loop.
To make it more obvious to the user that they have quit, we can add the following print statement outside of the loop.
print("Quitting...")
The code should look like this:
print("Operations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
quit = 'n'
while quit == 'n':
user_input = int(input("Make a Selection: "))
if user_input == 1:
print(str(user_input) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " + " + str(y) + " = " + str(x + y))
elif user_input == 2:
print(str(user_input) + ". Subtraction")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " - " + str(y) + " = " + str(x - y))
elif user_input == 3:
print(str(user_input) + ". Multiplication")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " * " + str(y) + " = " + str(x * y))
elif user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
quit = input("Quit(y/n)? ")
print("Quitting...")
Be sure to review and test your code.
Try to calculate some values using each operation without restarting (quitting).
Error Handling
The final part of the algorithm is error handling. Handling “bad” inputs.
- The program should handle ‘ValueErrors’ and bad inputs
- i.e. providing an error message if the user enters a string instead of an int without the program crashing.
What happens if a user tries to enter a value that wasn’t handled? The program will crash because it does not know what to do. Wait, what does handling values mean? Handling refers to certain conditions applied to data that the program will process. In this context of our calculator program, python handles the user inputs. In total there are 4 selections that can be made in our program, addition, subtraction, multiplication, and division. What happens if the user tries to enter 5
when selecting an operation? Well there is no fifth operation so the program will crash and python will throw an error. In other words when running the program, python does not know how to handle an input of 5
when selecting an operation.
This is a simple problem that can be fixed by adding an else
condition after the last elif
condition.
elif user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
else:
print("error: Invalid Input")
Now if the user enters a value that doesn’t correspond with the different operations (the user enters a value greater than 4) the else condition will fire and print "error: Invalid Input"
.
Now let’s do some testing an enter a value that is not an integer (a value that is non-numeric). If you do this you will get another error, ValueError: invalid literal for int() with base 10
. This error was thrown because our python program is expecting an integer from the user. If user the enters a
or %
or anything else non-numeric, then the program will crash and throw a ValueError
.
Ok, so now what? You could write a bunch of conditions to check to see if each value entered is an integer or you can use a try, except
block:
print("Operations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
quit = 'n'
while quit == 'n':
try:
user_input = int(input("Make a Selection: "))
if user_input == 1:
print(str(user_input) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " + " + str(y) + " = " + str(x + y))
elif user_input == 2:
print(str(user_input) + ". Subtraction")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " - " + str(y) + " = " + str(x - y))
elif user_input == 3:
print(str(user_input) + ". Multiplication")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " * " + str(y) + " = " + str(x * y))
elif user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
except ValueError:
print("Error: Invalid Input. Try again...")
quit = input("Quit(y/n)? ")
print("Quitting...")
Above is the program with the try/except
block implemented.
Try
allows the programmer to test code while it is being executed. Everything in the try
will be tested by python. Python will watch for errors.
Except
allows the programmer to define a block of code to be executed if an error occurs. Python is watching for errors in the try
block. If an error occurs python will run the code inside of the except
block instead of crashing our program and throwing an error. The only thing is we have to to tell python what to watch for.
Refer back to the error message ValueError: invalid literal for int() with base 10
. This is what we will tell python to watch for, so the except
block will look like except ValueError
. Now our program can handle “bad” inputs and will ask the user to try again if something other than an integer is entered.
Therefor
else:
print("error: Invalid Input")
can be removed, because now our code will watch for any values that are not compatible with our conditions.
Tweaks
Congrats! You made it through this tutorial.
Before moving on, let’s make some tweaks.
On the very first line, let’s print a title.
print("Simple Calculator program")
This will be the first thing the user sees when they launch the calculator program.
Next, let’s add the operation list inside of the while
loop.
quit = "n"
while quit == "n":
print("Operations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
...
If the user does a lot of calculations the list of operations may go out of view. To keep them from having to scroll back up we can reprint operations when the program restarts. Now every time the user starts a new calculation they will see operations.
The output looks a little crammed. Let’s give the text some room to breathe using \n
.
\n
stands for “next line.” In many programming languages you can use special characters to tell your console to do special actions when reading printed values back to the user. \n
tells the python to print on the next line.
To do something similar you could write two print statements:
print("hi")
print("there")`
Try it yourself. Compare:
print("Hello \nThere")
vs
print("Hello")
print("There")
In most cases, integrating \n
into your string is a lot faster and cleaner. It ultimately comes down to preference.
Placing \n
before “Operations:” will print the string on the next line:
print("\nOperations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
In addition, every time a calculation is completed and the user opts to do another calculation it will print all of operations on a new line creating some space between each calculation. This will make our program more readable for the user.
Try it out for your self On your own. Your output should match the example below:
#example output:
Simple Calculator Program
Operations:
1. Addition (+)
2. Subtraction (-)
3. Multiplication (*)
4. Division (/)
Make a Selection: 1
1. Addition
input first number: 2
input second number: 2
2 + 2 = 4
Quit(y/n)? n
Operations:
1. Addition (+)
2. Subtraction (-)
3. Multiplication (*)
4. Division (/)
Make a Selection: 3
3. Multiplication
input first number: 2
input second number: 2
2 * 2 = 4
Quit(y/n)? y
Quitting...
Now do the same for Make a Selection
and Quit(y/n)
.
As a final tweak, we can declare x
and y
at the start of our program, making these variables global. Global variables can be accessed anywhere in the code. Local variables are variables that are declared inside blocks of code. The advantage of creating a global variable is that we can store the variable in python’s memory early on. When we call it later, python remembers that it already read that variable and doesn’t have to spend more time creating it. When x
and y
is referenced after the user has made a selection, the variable will hold new values rather than python recording the user’s input and then storing it in a new variable. It essentially replaces the value that was already being held in that variable.
Here’s what your final code should look like:
print("Simple Calculator Program")
quit = 'n'
x,y = 0,0
while quit == 'n':
try:
print("\nOperations:")
print("1. Addition (+) ")
print("2. Subtraction (-) ")
print("3. Multiplication (*) ")
print("4. Division (/) ")
user_input = int(input("\nMake a Selection: "))
if user_input == 1:
print(str(user_input) + ". Addition")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " + " + str(y) + " = " + str(x + y))
elif user_input == 2:
print(str(user_input) + ". Subtraction")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " - " + str(y) + " = " + str(x - y))
elif user_input == 3:
print(str(user_input) + ". Multiplication")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " * " + str(y) + " = " + str(x * y))
elif user_input == 4:
print(str(user_input) + ". Division")
x = int(input("input first number: "))
y = int(input("input second number: "))
print(str(x) + " / " + str(y) + " = " + str(x // y))
except ValueError:
print("Error: Invalid Input. Try again...")
quit = input("\nQuit(y/n)? ")
print("Quitting...")
Now you can show off your sweet calculator program. Congrats!
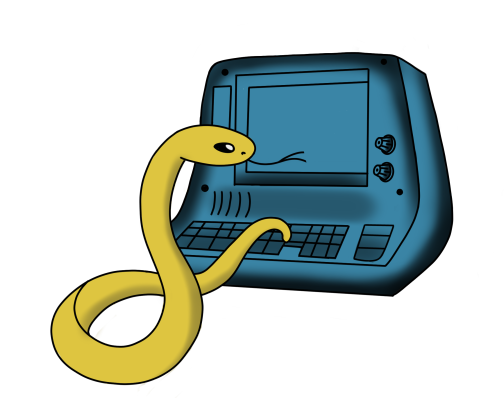