Published: 2024-06-22
Lesson 1 - Getting Started
Time To Complete: 2 hours
Getting Started
To start, you will need a development environment so you can write and run your code. There are several online platforms that will allow you to write and run code in your browser. Not installations required.
Wandbox
- Head over to wanbox.org
- On the left you will see a list of programming languages. Scroll down until you find “python”
- Click on “python” and that’s it. You’re ready to start writing code.
python-online
- Head over to python-online.com
- That’s it. You’re ready to start writing code.
wandbox vs. python-online
The downside of using python-online is there are a lot of ads. To remove these ads, you can add an ad blocker to your browser extensions. I recommend uBlock.
Wandbox is ad free, but some programs that you will write in this course will require python-online. This is because wandbox is just a compiler. It is not interactive. Some lessons will require an Integrated Development Environment (IDE). python-online is an IDE.
Hello World
If we want to display text in python we have to wrap our words in quotations.
If we don’t, python will think we are trying to give it some sort of special instructions.
print("Hello World!")
- To run your code click the “run” btn.
Variables
Variable is just a label that represents an object in a computer program.
x = 100
We use the equal sign to create a variable.
The name of a variable can be anything .
I can use ‘a’ or ‘b’ or ‘asdf’ it does not matter what I call it as long as I provide an equal sign and a value after the equal sign.
show the value of x using the print() function and change the value of x
print(x)
x = 200
print(x)
we can also create a new variable independent of x.
y = x
print(y)
we just told python that we want to label y and x as the same thing.
print(y)
print(x)
Python reads them as the same value, because we told it to do that.
Let’s use a name more useful
number_of_coins = 200
print(number_of_coins)
Variables can use letters, numbers and underscores.
- They cannot start with numbers
- Names should be meaningful
- in some situations it’s ok for them to be short.
Experimenting with Strings, Ints and the print() function.
In python we use the print()
to show information in the console.
We can use print to show display all kinds of data.
print(444)
number = 3
print(number)
name = "fred"
print(name)
greeting = "Hello World"
We can even print multiple items at the same time by using the +
or by separating values using ,
.
print(444 + number)
print(4 * number)
print(greeting + "!")
print(greeting , name)
#console output -> "Hello World , Fred"
Take note of the last item we printed.
We used a comma instead of a plus sign.
print(greeting + name)
Notice how the words are all crammed together now.
When we use the plus sign we tell python to concatenate or combine them.
If we use numbers we get a sum, if we use words python combines them together.
When we use a comma we tell python to display both of them at the same time rather than combining them.
Coin Exercise
A local wizard can create gold coins from coal. You already have found 20 coins. You bring him a big lump of coal that he turns into 10 gold coins every day for an entire year.
There is an evil crow that terrorizes you on your walks home from the wizard’s place. The crow manages to steal 3 coins from you a week.
If there are 365 days in a year and 52 weeks in a year, how many coins will you have at the end of the year?
found_coins = 20
magic_coins = 10
stolen_coins = 3
total = found_coins + magic_coins * 365 - stolen_coins * 52
print("my life savings: " + total)
##3514
What if you wore a scarecrow outfit to scare off the crow
and now you only lose 2 coins a week. What would you change?
stolen_coins = 2
What if you only started with 13 coins instead of 20?
found_coins
Strings
Label a string
fred = "Hello my name is Fred."
Create a string using more than one line
#incorrect
anti_joke = "why did the chicken cross the road?
To get to the other side."
#SyntaxError: EOL while scanning string literal
- Syntax refers to an order that the python compiler expects.
- SyntaxError means the user provided an order the compiler was not expecting.
#correct
anti_joke = '''why did the chicken cross the road?
To get to the other side.'''
Handling Problems with String
silly_string = 'He said, "Aren't can't shouldn't wouldn't."'
We used a mixture of quotes that python could not understand.
- You have to be careful with single and double quotes.
- when python sees a bunch of quotes , its expecting strings NOT a bunch of punctuation.
Solution to this problem is a multiline string.
silly_string = '''He said "Aren't can't shouldn't wouldn't."'''
The multiline strings tells python “hey, ignore all of the quotes in this string. Print them as is.”
We can also get around this issue using escape characters
single = 'He said, "Aren\'t can\'t shouldn\'t wouldn\'t."'
double = "He said, \"Aren't can't shouldn't wouldn't.\""
- notice how the backslash does not show
- this is simply another method, in most cases multiline is a lot easier to read.
Embedded Strings
This is a method of displaying a message in combination of a variable.
- You can embed values using
%s
.%s
is a marker that you want to use later.
- Allows you to insert the value of a variable into a string easily.
myscore = 1000
message = 'I scored %s points'
print(message % myscore)
You can also use more than one placeholder in a string.
message = 'I scored %s points out of %s'
print(message % (800, 1000))
the order of the embedded values matters
Multiplying Strings
What is 10 x 5?
What about 10 x a?
print(10 * "a")
print(1000 * "a")
Fighting Fighters
If there are 3 buildings with 25 ninjas hiding on each roof and 2 tunnels with 40 samurai hiding inside each tunnel, how many ninjas and samurai are about to do battle?
samurai = 2 * 40
ninjas = 25 * 3
total = samurai + ninja
print(total)
Greetings
Create two variables: one that will hold your first name and one that that will hold your last name. Now create a string and use embedded placeholders to print your name with a message using those two variables, such as “Hi there, Brando Ickett!”
greeting = "Hi there, %s %s"
print(greeting % (Landon, Rickets))
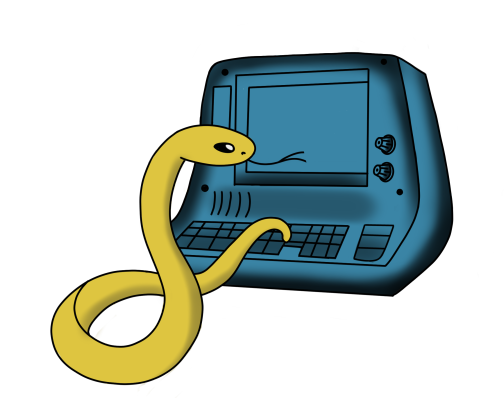