Published: 2024-07-13
Lesson 3 - Loops
Time To Complete: 2 hours
Programmers are lazy.
Writing the same commands over and over again can get boring.
Most programming languages have tools referred to as loops.
A loop is a block of code that repeats other programming statements automatically.
Using For-Loops
print hello world five times without using copy(ctrl+c) paste(ctrl+v).
print("hello world")
print("hello world")
print("hello world")
print("hello world")
print("hello world")
I didn’t even bother typing that 5 times. I used copy paste…
Now, let’s print hello world 5 times while only writing 1 print statement using a “for loop.”
for i in range(0,5): #line #1
print("hello world") #line #2
Awesome, but what does it all mean?
The range function on line 1 tells python to start counting from x and stop before y, in this case start counting from 0 and stop before 5.
Reading a for loop:
FOR a value in range 0 to 5, count and store the value in
i
.
Python reads the for loop at line 1
, then executes the block of code at 2
. “Hello world” will print 5 times because our loop tells python to run line 2
on each iteration the value of i
increase.
If you want to experiment with the range function to better understand how it works, you can print a range as a list. The list will contain values within the range.
print(list(range(0,5)))
you can also use the i
in our print statement to count each “hello world.”
for i in range(0,5):
print('hello world %s' % i)
Remember python starts counting from 0. It’s a zero-indexed language.
If you want to do the same thing without using a “for loop” your code might look like this.
x = 0
print('hello %s' % x)
hello 0
x = 1
print('hello %s' % x)
hello 1
x = 2
print('hello %s' % x)
hello 2
x = 3
print('hello %s' % x)
hello 3
x = 4
print('hello %s' % x)
hello 4
Good programmers are lazy. They find ways to solve problems with as few lines of code as possible.
Looping through Collections
You don’t have to use a range function to iterate through a loop.
You can also use a data structure such as a list!
Think back to the grocery list in lesson2.
food_list = [
'bread',
'butter',
'milk',
'eggs',
'cheese'
]
for item in food_list:
print(item)
This loop is another way of saying “FOR EACH item in the food_list, store the value in variable item
and then print contents.
Another way to say it is “FOR EACH item in the food_list, print the item”.
If you wanted to print each item without using a loop, you would have to write something like:
print(food_list[0])
print(food_list[1])
print(food_list[2])
print(food_list[3])
print(food_list[4])
Using a for loop saves you a lot of typing.
Now, run the following code.
words = ['first','second','third']
for word in words:
print(word)
print(word)
Looking at the code, you might expect the first and second item to be printed.
In reality it prints the first item twice. Then the second item twice and then the third item twice. This is because you are looping through the list.
On the first iteration you’ll be at index 0 which is ‘first’.
Next, index 2 which is ‘second’. Finally, index 3 which is ‘third’.
After the 3rd iteration, the loop stops and python moves on, except there is no more code so python closes the program.
Let’s code a for loop with two blocks of code.
words = ['first','second','third']
for word in words:
print(word)
#char is short for character
for char in word:
print(char)
This is a nested for loop, a loop within a loop. The first block is the first for
loop(the outer loop) and the second block is the second for
loop(the inner loop).
The string at index word
will print, then the loop will print each char
in the word.
Let’s try another nested for loop.
words = ['first','second','third']
for i in words: #outer loop
print(i)
for j in words: #inner loop
print(j)
print("\n")
In the code above, python enters the first loop, the outer loop, and prints an item from the list using the print(i)
statement. Next, if enters the second loop, the inner loop, and prints all items in the list with the print(j)
statement. It then exits the inner loop and continues to the next iteration of the outer loop until the there are no more items in the list.
What is i
and j
about?
Perviously, you had used “word” and “char” to represent items within our list. Recall that when you create a loop you use a variable to temporarily store a given value at a given iteration. The variable used to store values within the loop can be named anything. If you are looping through a list of words, you can write the loop like this for word in words:
like you have done before. If you want, you can make something up like so for asdf in words:
. Often times programmers just use i
, because they are lazy. i
may stand for “iteration” because a loop iterates, or it could just mean “i” and nothing more. In the nested for loop above, “j” is used because it is the next letter in the alphabet.In short, i and j are not used for anything special, they are just common variable names programmers use.
Revisiting the coin problem
Recall in lesson 1, you met a wizard that could create gold coins from lumps of coal.
The variables in that problem were:
- found coins = 20
- magic coins = 70 , because 10 coins a day * 7 days in a week equal 70
- stolen coins = 3 Let’s solve this problem using a for loop this time
found_coins = 20
magic_coins = 70
stolen_coins = 3
coins = found_coins #1
for week in range(1,53): #2
coins = coins + magic_coins - stolen_coins #3
print('Week %s = %s' % (week,coins)) #4
At 1
, the variable coins
is loaded with the value of the variable found_coins
; this is what you start with.
2
sets up the for loop, which will run all of the code in the block (the block is made up of lines 3 and 4). Each time it loops, the variable week
is loaded with the next number in the range of 1 to 52. Wait, in the code the loop uses range(1,53)
. Right, the range function in python is EXCLUSIVE, so it will count values from 1 UP TO but not including 53. Meaning the loop only runs 52 times, the number of weeks in a year.
3
adds the number of coins the wizard magically created and subtracts the number of coins the crow stole. The variable coins
represents our total. It acts like a wallet or treasure chest where you would store all your coins. Every week, new coins are piled into the chest. What 3
is telling python to do is to compute how many coins you have on the right side of =
and save it for later using the variable name on the left side of the =
.
4
prints how many coins you have at the end of each week using placeholders.
Printing squares
Let’s think about geometry for a sec. A square is a shape where the length and the width is equal. If length and width are not equal, then it is a rectangle. How can you use use for loops to print a picture of a square? Using a nested for loop.
#drawing a 3x3 square
for i in range(3):
piece = ""
for j in range(3):
piece += "x"
print(piece)
Let’s break this problem down. For a 3x3 square, like in the code above, you can think of a square as a series of columns and rows. You could print a square like so:
print('''
xxx
xxx
xxx''')
but you want to draw a square using loops. Look at the print statement. There a rows and columns. 3 rows and 3 columns to be exact. Using a loop, you would loop through a row with one loop and then loop through each column using another loop before starting the next row. To draw the square you can use a string. You can use the two for loops to build the string. That might feel a bit overwhelming, but that’s ok. This is a problem that is tricky to think about, but really the code is not as complicated.
Let’s look at the code again.
#drawing a 3x3 square
for i in range(3): #1
piece = "" #2
for j in range(3): #3
piece += "x" #4
print(piece) #5
Line By Line
Starting at 1
, we set up the outer loop. This outer loop will represent the rows in the square. If you are drawing a 3x3 square, there will be 3 rows and 3 columns. On the first iteration, python will build the first row, the second iteration the second row and so on.
On 2
we create the string variable piece
, which is set to an empty string. That’s what ""
means. It is empty because as we iterate through the second loop we will add the "x"
’s which will represent the columns.
Line 3
sets up the second loop. This loop will represent the columns in our square. Python will iterate through the loop 3 times because you need 3 columns.
4
is where the string is built. Notice the +=
. This operation is called an incrementor. Every time python iterates through the second loop it increments the value of piece
by one "x"
.
5
prints piece at the end of the each iteration of the outer for loop, the loop on line 1
.
Putting It All Together
- Python begins iterating through the outer for loop at
1
, the first iteration represents the first row. - The
piece
string variable is initialized(another word for “set” or “created”) as an empty string at2
. - Python then starts iterating through the inner for loop at
3
where it will iterate 3 times to build 3 columns. - On each iteration python will increment
piece
by 1"x"
at line4
. - Finally at
5
, python will printpiece
which will displayxxx
for that row. - The next iteration creates a new row and the code continues until 3 rows with 3 columns have been created.
This is a lot of information. If you don’t get quite get it yet that is perfectly fine. If you do, awesome. Either way keep practicing and you will get to really understand for loops, which are very useful and important in programming.
It is important to practice and understand for loops as they can be challenging for beginners. The hard part about loops is that you have to really think about what is happening behind the scenes. If you know what’s coming next in your loop and you run into issues, it makes debugging far easier.
For Loops in Different Languages
In time for loops will become second nature. If you start using other languages like Java, C++, or Javascript you’ll find writing for loops requires a few more lines of code, but you won’t have to re-learn what a loop is. The only thing you’ll have to learn of is the syntax.
Java
//print numbers 1 through 4
for (int i = 0; i < 5; i++){
System.out.println(i);
}
Javascript
//print numbers 1 through 4
for (let i = 0; i < 5 ; i++){
console.log(i);
}
C++
for (int = 0 ; i < 5; i++){
cout << i << "\n"
}
As you can see, in the above 3 languages the for loop is quite similar. Python spoils us with less syntax. How nice.
While Loops
A for
loop isn’t the only kind of loop. In programming there are definite iterations, where the number of repetitions is specified explicitly in advance and there are indefinite iterations where the code block executes until some condition is met.
A for
loop is used when there is a specified length. You have a range or a collection, such as a list that you are looping through. A while
loop is a loop that is used when you don’t know ahead of time when it needs to stop. The while
loop will stop when some condition is met.
Imagine a staircase with 20 steps. The staircase is indoors. You can climb that easily.
Here’s what a for
loop for that looks like:
for step in range(0,20):
print(step)
Now imagine a staircase on a mountain, like the Manitou incline in Manitou Springs. CO. There’s a staircase, but the climb is about 2000 feet. You might run out of energy, or there might be bad weather that forces you to stop.
Here’s what a while
loop for that looks like:
step = 0
while step < 2500:
print(step)
if tired == True:
break
elif badweather == True:
break
else:
step += 1
Great, except if you run this code you will get an error. tired
and badweather
have not been defined yet. If we set badweather
and tired
to False
, then the program will run until steps equal 2500.
tired, badweather = False, False
step = 0
while step < 2500:
print(step)
if tired == True:
break
elif badweather == True:
break
else:
step += 1
print("2500!")
Programming Problems
Hello loop
What do you think the following loop will do?
for x in range(0,20):
print('hello %s' % x)
if x < 9:
break
Try to guess what will happen, then run it yourself to see if you were right.
Favorite Food List
Create a list containing your five favorite foods.
Example:
fav_foods = ['Pizza', 'French Fries', 'Chicken Tikka Masala','Poke', 'Chicken parm']
Now create a loop that prints out the list (including numbers):
fav_foods = ['Pizza', 'French Fries', 'Chicken Tikka Masala','Poke', 'Chicken parm']
num = 0
for food in fav_foods:
num+=?
#code the rest
#output:
1 Pizza
2 French Fries
3 Chicken Tikka Masala
4 Poke
5 Chicken Parm
Weight of an Object on the Moon
There is a bucket of rocks that weighs 145 pounds. If 1 lb of rocks is added every year, how much would the bucket weigh on the moon after 15 years?
hint To get the weight of an object on the moon simply multiply
weight * .165
.
hint: this one is similar to the coin problem with the for loop.
weight = 145
moon = .165
added = 1
for i in range(1,16):
weight += ?
moon_weight = ?
#code the rest
Puzzle Solutions
Favorite Food List
fav_foods = ['pizza', 'french fries', 'chicken tikka masala','poke', 'chicken parm']
i = 0
for f in fav_foods:
i+=1
print('%s %s' % (i,f))
Weight of an Object on the Moon
weight = 145
moon = .165
added = 1
for i in range(1,16):
weight += added
moon_weight = weight * moon
print('year %s: %s' % (i,moon_weight))
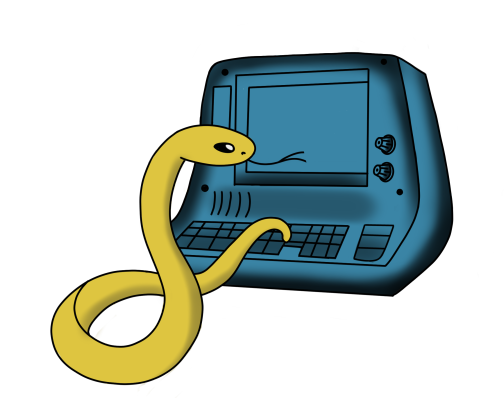